Variables & Functions
A variable is a named value that references or stores a piece of data. Variables can be assigned by using an “=” sign, as shown below:
Variable names must start with a letter and cannot contain special characters.
Variables can be updated with operations and can assume different data types. The operations available to each variable differ based on data type--for instance, you can't divide a "True" by "False"!
Statements & Expressions
An expression is a combination of symbols that represent a value. Examples:
Statements are lines of code that perform actions. Statements may be made up of expressions. Examples:
Functions
A function is a named part of a program that performs some procedure. The format in which we declare functions in Python is shown below.
We can see that functions are made up of three elements: a name, parameters, and some action (the body). The header is made up of the name and parameters. We separate the header and body with a newline and an indent--most Python programmers use tabs to indent.
Note that functions don't necessarily need to have any parameters at all! This is done if nothing is included between the parantheses.
A return statement, well, returns a value as part of a function. This ends a function immediately. If a function has no return statement, it will return None. An example of a function that multiples two numbers:
A lot of newbies in Python will confuse print() and return. print() is a function that shows, in the console, what you put between the brackets. return is just a statement, so you won't see it unless you print it. For example:
Local vs. Global Variables
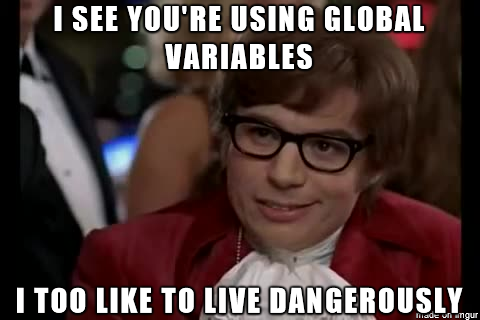
Variables are defined within a specific scope--this means that they can't be used everywhere in your code, but only where you define them.
A local variable is one that is defined inside a function, and hence only exist inside that function. An example:
A global variable is the complete opposite--it is declared outside functions, and can be used anywhere. Usually, it is good style to not use global variables, because it would "pollute the global namespace", making it difficult to keep track of the scope of variables.
Helper Functions
You can call functions inside other functions! These are typically called helper functions, and are quite nice in implementing more advanced algorithms.